Problem : Write pseudocode for a non-recursive version of an inorder traversal of a binary search tree. Assume the nodes have pointers to their parent nodes. (Hint: for any node, where is the node with the next highest value?)
- start at the leftmost node (the smallest)
- while there is a current node
- process that node
- find the next smallest node (the inorder successor):
- if the current node has a right child, set the next node to the leftmost node in the right subtree
- otherwise, set the next node to deepest ancestor of the current node so that the path from that ancestor to the current node follows a left branch (back up the tree until you've backed up a left branch)
- set the current node to be the next node
Problem : Draw the binary search tree that results from adding SEA, ARN, LOS, BOS, IAD, SIN, and CAI in that order.
SEA / \ ARN SIN \ LOS / BOS \ IAD / CAI
Find an order to add those that results in a tree of minimum height.
IAD, BOS, ARN, CAI, SEA, LOS, SIN
Find an order to add those that results in a tree of maximum height.
SIN, SEA, ARN, LOS, BOS, IAD, CAI
For your original tree, show the result of removing SEA, then show the order in which the nodes would be processed by an inorder traversal.
SIN / ARN \ LOS / BOS \ IAD / CAIor
LOS / \ ARN SIN \ BOS \ IAD / CAI
ARN, BOS, CAI, IAD, LOS, SIN
Problem : Repeat the original adds and delete from the previous problem for an AVL tree.
LOS / \ BOS SEA / \ \ ARN IAD SIN / CAIAfter all deletes:
IAD / \ BOS LOS / \ \ ARN CAI SIN
Problem : Repeat the original adds from the previous problem for a Red-Black tree.
LOS / \ BOS SEA / \ \ ARN IAD SIN / CAI
Problem :
Consider the remove_incoming
operation, which takes a
vertex $v$ in a directed graph and removes all incoming edges to that
vertex. Explain how to implement remove_incoming
when the
graph is represented using an adjacency matrix and then for an adjacency list.
What is the asymptotic running time of your implementations in terms of
the number of vertices $n$ and the total number of edges $m$?
For an adjacency matrix: set all entries in the column for vertex
$v$ to false
. This will take $\Theta(n)$ time.
for each vertex u use sequential search to find v on u's adjacency list if found, deleteThis will take $\Theta(n+m)$ time since in we iterate over all entries in the adjacency lists (worst case if the adjacency lists are linked lists because we can stop when we find $v$; every case if the adjacency lists are arrays and we want to preserve the order of the adjacency list : we would go through the array to find $v$ and then go through the rest of the array to move elements back to fill the hole created when $v$ was removed).
Problem : Show the result of running breadth-first search on the following directed graph. Start at vertex $a$ and after dequeueing a vertex, consider its neighbors in alphabetical order.
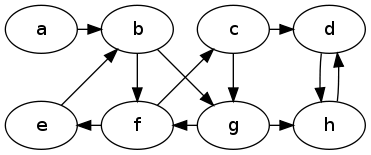
pred[v] = u
then v
is a child of u
in the tree
d=0 a | d=1 b / \ d=2 f g / \ \ d=3 c e h | d=4 d
Problem : Show the result of running depth-first search on the graph in the previous problem. Start at vertex $a$ and when you get to a vertex, consider its neighbors in alphabetical order.
a | b | f / \ c e / \ d g | h